An observation on .NET loops – foreach, for, while, do-while
Posted
on Microsoft .NET Support Team
See other posts from Microsoft .NET Support Team
Published on Mon, 24 Jan 2011 11:49:00 +0000
Indexed on
2011/01/28
23:34 UTC
Read the original article
Hit count: 282
Filed under:
It’s very common that .NET programmers use “foreach” loop for iterating through collections. Following is my observation whilst I was testing simple scenario on loops. “for” loop is 30% faster than “foreach” and “while” loop is 50% faster than “foreach”. “do-while” is bit faster than “while”. Someone may feel that how does it make difference if I’m iterating only 1000 times in a loop.
This test case is only for simple iteration. According to the "Data structure" concepts, best and worst cases are completely based on the data we provide to the algorithm. so we can not conclude that a "foreach" algorithm is not good. All I want to tell that we need to be little cautious even choosing the loops.
Example:- You might want to chose quick sort when you want to sort more numbers. At the same time bubble sort may be effective than quick sort when you want to sort less numbers.
Take a simple scenario, a request of a simple web application fetches the data of 10000 (10K) rows and iterating them for some business logic. Think, this application is being accessed by 1000 (1K) people simultaneously. In this simple scenario you are ending up with 10000000 (10Million or 1 Crore) iterations.
In above example, I was just iterating through 100 Million numbers. You can see the time to execute various loops provided in .NET
Output
"foreach" took 1108 milliseconds
"for" loop took 727 milliseconds
"while" loop took 596 milliseconds
"do-while" loop took 594 milliseconds
Press any key to continue . . .
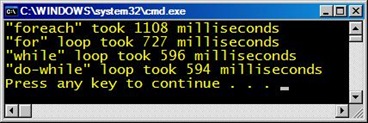
So I feel we need to be careful while choosing the looping strategy. Please comment your thoughts.

This test case is only for simple iteration. According to the "Data structure" concepts, best and worst cases are completely based on the data we provide to the algorithm. so we can not conclude that a "foreach" algorithm is not good. All I want to tell that we need to be little cautious even choosing the loops.
Example:- You might want to chose quick sort when you want to sort more numbers. At the same time bubble sort may be effective than quick sort when you want to sort less numbers.
Take a simple scenario, a request of a simple web application fetches the data of 10000 (10K) rows and iterating them for some business logic. Think, this application is being accessed by 1000 (1K) people simultaneously. In this simple scenario you are ending up with 10000000 (10Million or 1 Crore) iterations.
below is the test scenario with simple console application to test 100 Million records.
using System;
using System.Collections.Generic;
using System.Diagnostics;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
var sw = new Stopwatch();
var numbers = GetSomeNumbers();
sw.Start();
foreach (var item in numbers)
{
}
sw.Stop();
Console.WriteLine(
String.Format("\"foreach\" took {0} milliseconds",
sw.ElapsedMilliseconds));
sw.Reset();
sw.Start();
for (int i = 0; i < numbers.Count; i++)
{
}
sw.Stop();
Console.WriteLine(
String.Format("\"for\" loop took {0} milliseconds",
sw.ElapsedMilliseconds));
sw.Reset();
sw.Start();
var it = 0;
while (it++ < numbers.Count)
{
}
sw.Stop();
Console.WriteLine(
String.Format("\"while\" loop took {0} milliseconds",
sw.ElapsedMilliseconds));
sw.Reset();
sw.Start();
var it2 = 0;
do
{
} while (it2++ < numbers.Count);
sw.Stop();
Console.WriteLine(
String.Format("\"do-while\" loop took {0} milliseconds",
sw.ElapsedMilliseconds));
}
#region Get me 10Crore (100 Million) numbers
private static List<int> GetSomeNumbers()
{
var lstNumbers = new List<int>();
var count = 100000000;
for (var i = 1; i <= count; i++)
{
lstNumbers.Add(i);
}
return lstNumbers;
}
#endregion Get me some numbers
}
}
In above example, I was just iterating through 100 Million numbers. You can see the time to execute various loops provided in .NET
Output
"foreach" took 1108 milliseconds
"for" loop took 727 milliseconds
"while" loop took 596 milliseconds
"do-while" loop took 594 milliseconds
Press any key to continue . . .
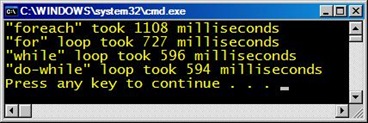
So I feel we need to be careful while choosing the looping strategy. Please comment your thoughts.
© Microsoft .NET Support Team or respective owner