Re-Centering JQuery Tooltips when resizing the window
Posted
by leeand00
on Stack Overflow
See other posts from Stack Overflow
or by leeand00
Published on 2009-09-14T16:27:07Z
Indexed on
2010/06/07
23:42 UTC
Read the original article
Hit count: 403
I have written a function that positions a tooltip just above a textbox.
The function takes two arguments:
textBoxId - The ID of the textbox above which the tooltip will appear.
- Example: "#textBoxA"
- Example: "#textBoxA"
toolTipId - The ID of the tooltip which will appear above the textbox.
- Example: "#toolTipA"
function positionTooltip(textBoxId, toolTipId){
var hoverElementOffsetLeft = $(textBoxId).offset().left;
var hoverElementOffsetWidth = $(textBoxId)[0].offsetWidth;
var toolTipElementOffsetLeft = $(toolTipId).offset().left;
var toolTipElementOffsetWidth = $(toolTipId)[0].offsetWidth;
// calcluate the x coordinate of the center of the hover element.
var hoverElementCenterX =
hoverElementOffsetLeft + (hoverElementOffsetWidth / 2);
// calculate half the width of the toolTipElement
var toolTipElementHalfWidth = toolTipElementOffsetWidth / 2;
var toolTipElementLeft = hoverElementCenterX - toolTipElementHalfWidth;
$(toolTipId)[0].style.left = toolTipElementLeft + "px";
var toolTipElementHeight = $(toolTipId)[0].offsetHeight;
var hoverElementOffsetTop = $(textBoxId).offset().top;
var toolTipYCoord = hoverElementOffsetTop - toolTipElementHeight;
toolTipYCoord = toolTipYCoord - 10;
$(toolTipId)[0].style.top = toolTipYCoord + "px";
$(toolTipId).hide();
$(textBoxId).hover(
function(){ $(toolTipId + ':hidden').fadeIn(); },
function(){ $(toolTipId + ':visible').fadeOut(); }
);
$(textBoxId).focus (
function(){ $(toolTipId + ':hidden').fadeIn(); }
);
$(textBoxId).blur (
function(){ $(toolTipId+ ':visible').fadeOut(); }
);
}
The function works fine upon initial page load:
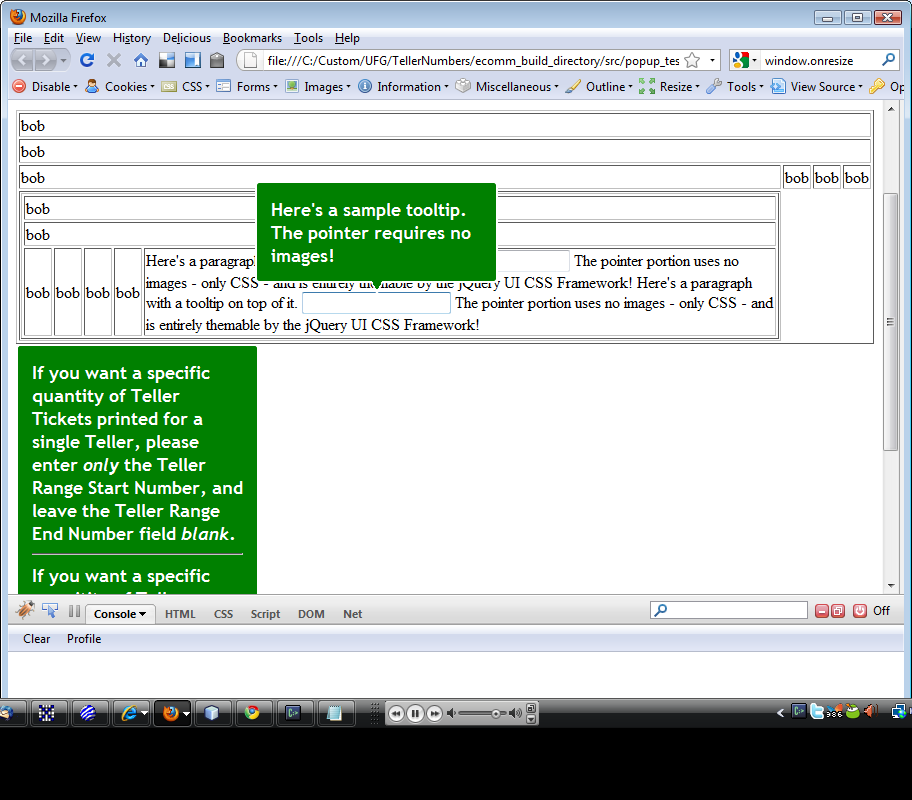
However, after the user resizes the window the tooltips move to locations that no longer display above their associated textbox.
I've tried writing some code to fix the problem by calling the positionTooltip() function when the window is resized but for some reason the tooltips do not get repositioned as they did when the page loaded:
var _resize_timer = null;
$(window).resize(function() {
if (_resize_timer) {clearTimeout(_resize_timer);}
_resize_timer = setTimeout(function(){
positionTooltip('#textBoxA', ('#toolTipA'));
}, 1000);
});
I'm really at a loss here as to why it doesn't reposition the tooltip correctly as it did when the page was initially loaded after a resize.
© Stack Overflow or respective owner